Using Keycloak to authenticate SynthAPI
This tutorial outlines a possible configuration and methodology used to authenticate client library sessions with a Keycloak instance running as part of a Hazy installation. It utilises the client credentials OAuth flow: note that this credential should be managed accordingly.
Keycloak setup¶
Keycloak is used as a third-party identity provider alongside the Hazy product for both authentication and authorisation. This tutorial assumes you have Hazy installation running with Keycloak active.
Here we'll add a new Keycloak Client called api
which is responsible for issuing token-based access to the resource server
Client hazy-hub-backend
. We use "Client Id & Secret" authentication in this example.
- In the Keycloak admin console, ensure you are in the "Hazy" realm, navigate to Clients and click Create Client. Here we'll
add a new Client called
api
with the Client IDapi
of type "OpenID Connect".
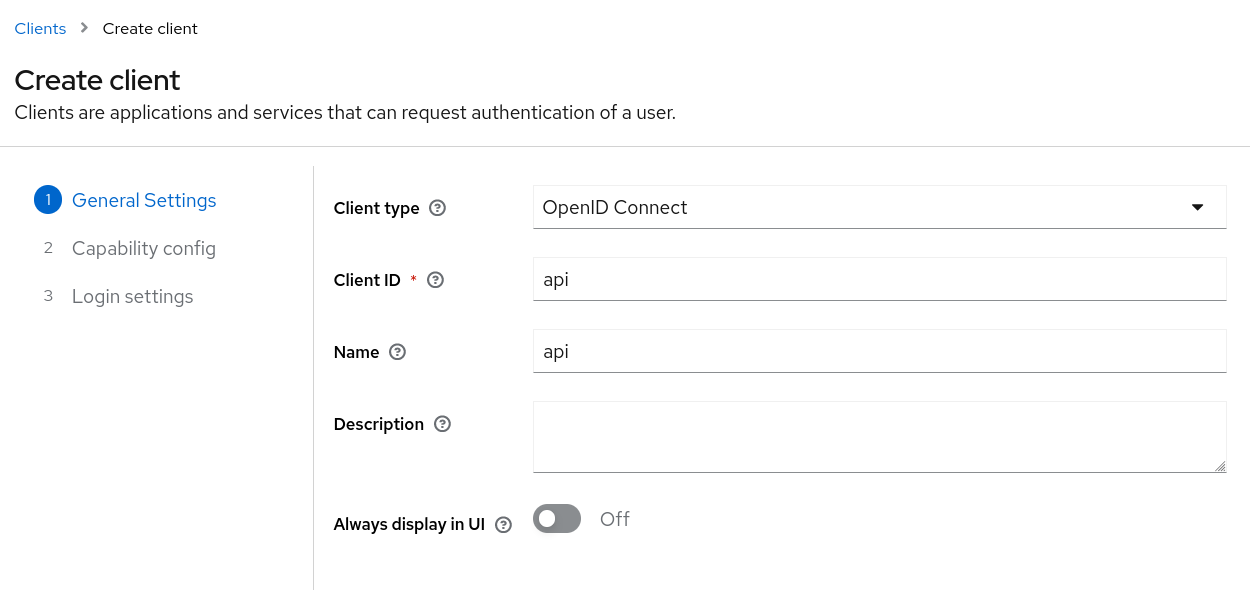
- Click "Next", and ensure "Client authentication" is enabled for this Client (leave the rest as default, including the "Login settings" pane). Confirm the Client to add to the Hazy realm.
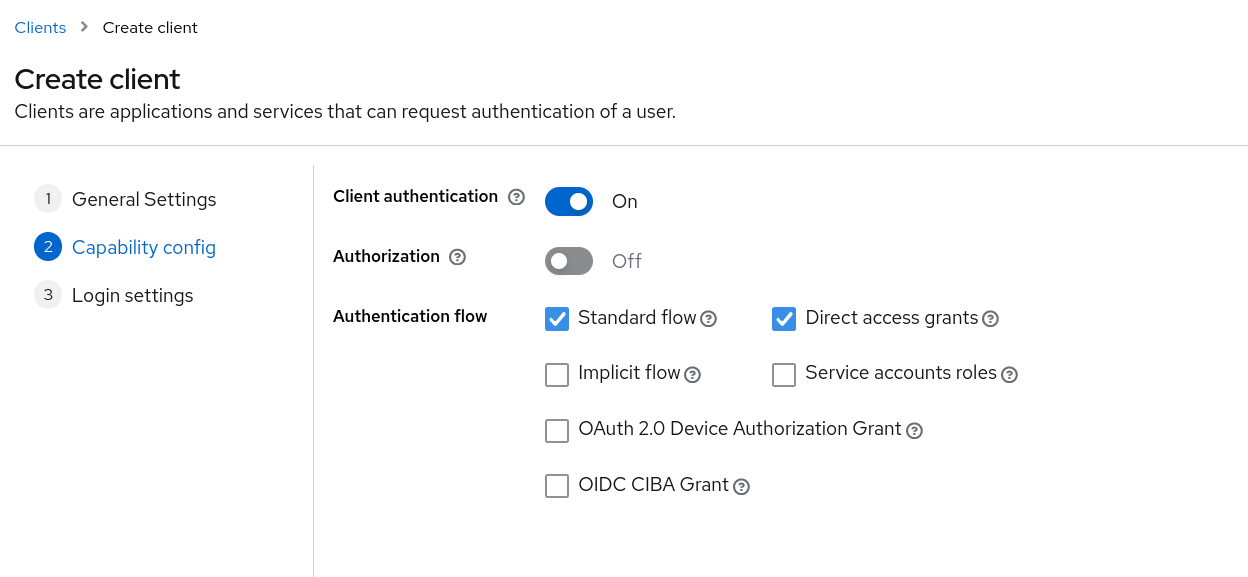
- With the
api
Client now available in the Client list, click through into its settings and select "Client Scopes" from the top tab.
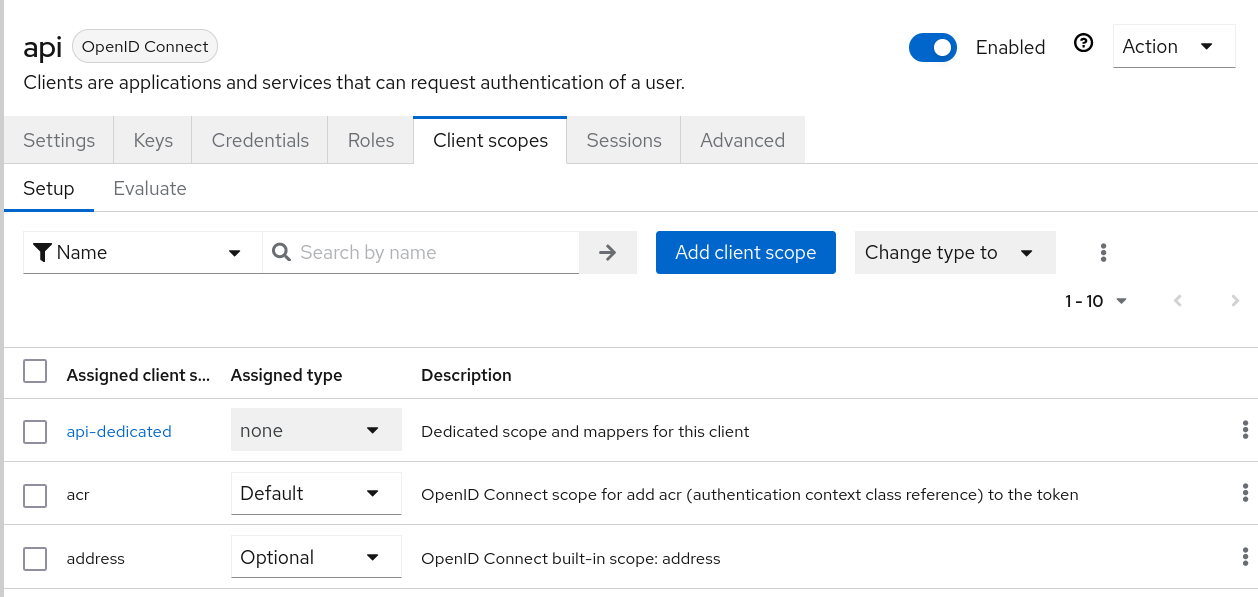
- Select the
api-dedicated
Assigned Client Scope, and then select "Configure a new mapper" from the next page.
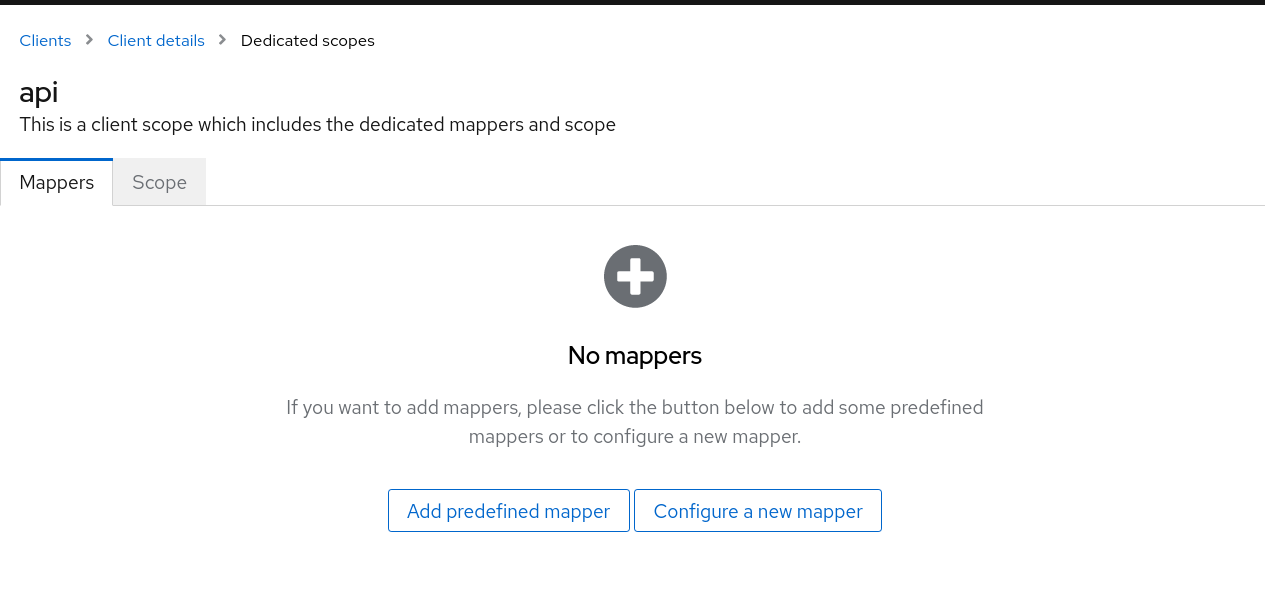
- Add an "Audience" mapper with the Included Client Audience value of
hazy-hub-backend
and "Add to access token" set to True. This mapper allows for OpenID passthrough to the resource server inhazy-hub-backend
when successfully auth'd against theapi
Client.
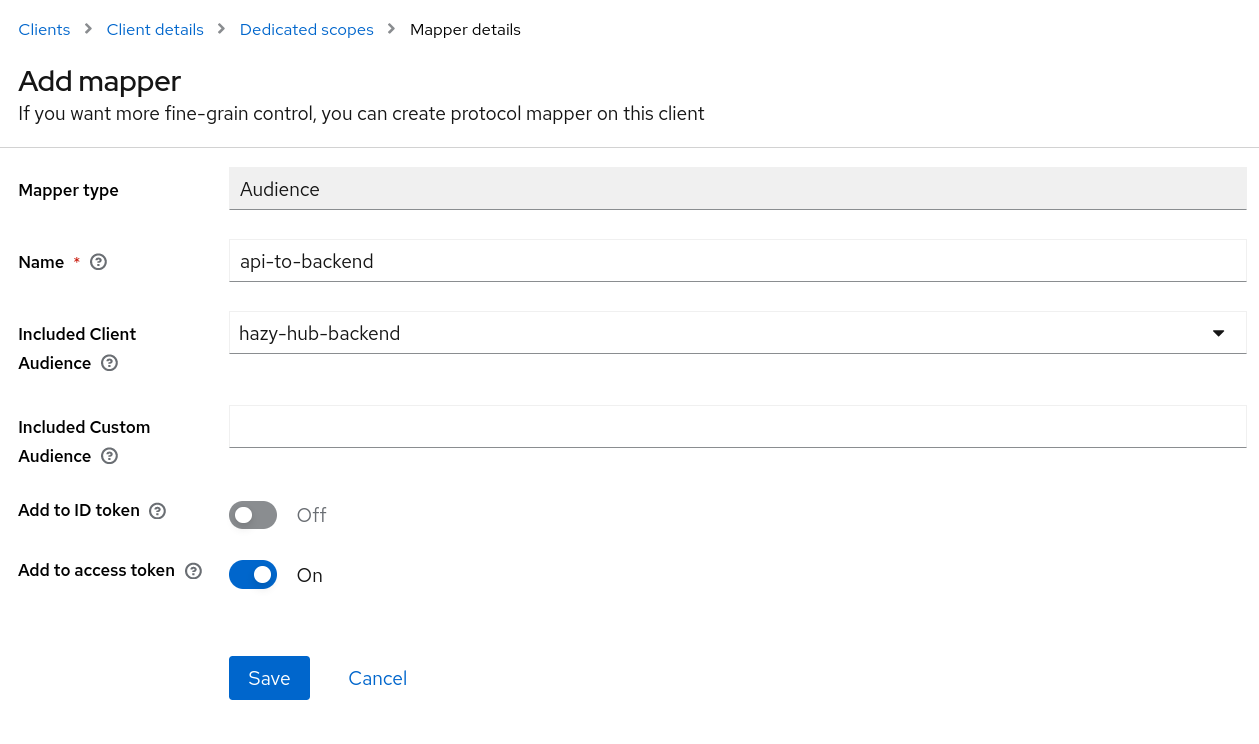
- Remaining on the Client Scopes page, ensure all requisite Hazy Client Scopes are added to the
api-dedicated
resource. For example, if you want the API to have the ability to train jobs then ensure thehazy/TrainJob:*
scopes are present here.
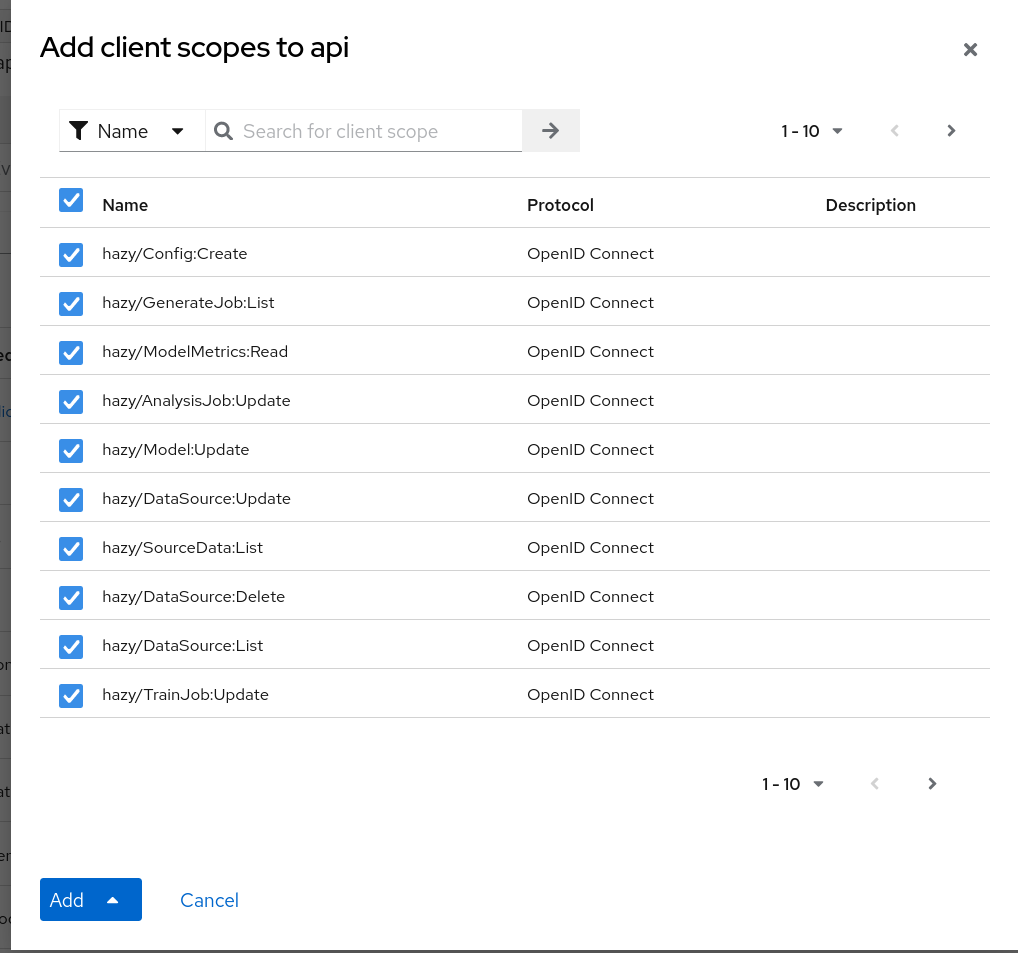
- Finally, make a note of the "Client secret" value from the Credentials tab of the
api
Client. This will be the API key used by the SDK to authenticate the OpenID Connect handshake and getSynthAPI
access to the Hub.
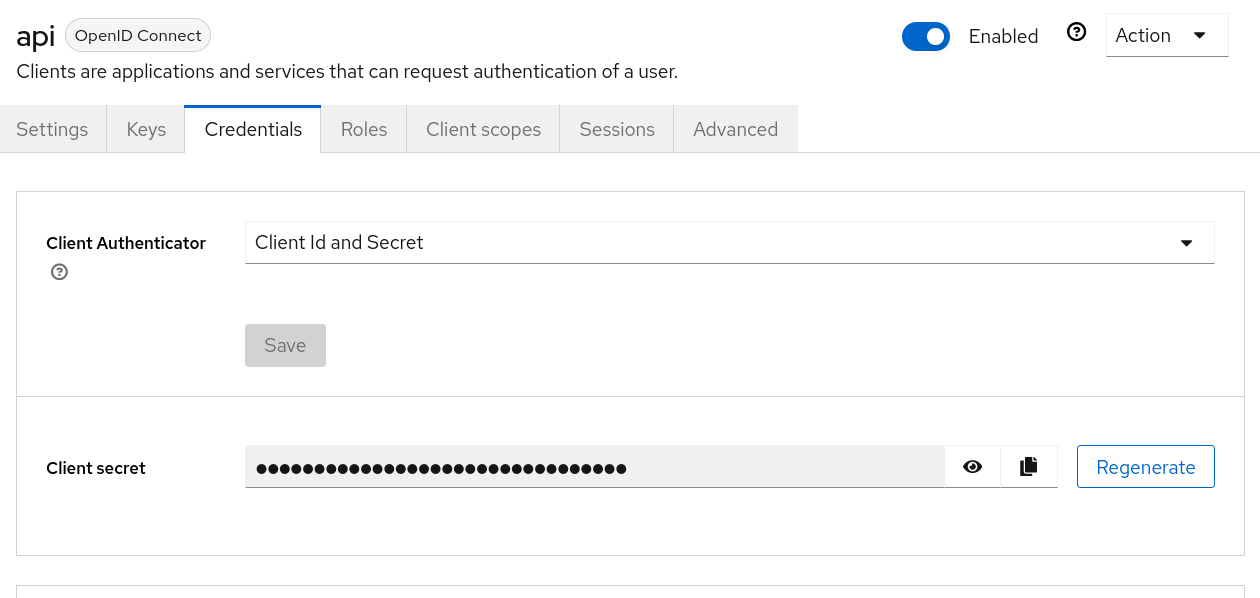
Authentication¶
With Keycloak configured as above, SynthAPI
can make requests to a running Hub instance using the Client Secret of the
API Client. A simple training example (with the requisite hazy_configurator
resources added) would look like:
import requests
from hazy_client2 import SynthAPI
HAZY_HUB_HOST = "https://your/hazy/hub"
params = {
"grant_type": "password",
"username": "...",
"password": "..",
"audience": "hazy-hub-backend",
"client_id": "api",
"client_secret": "...",
}
headers = {"content-type": "application/x-www-form-urlencoded"}
response = requests.post(
f"{HAZY_HUB_HOST}/auth/realms/hazy/protocol/openid-connect/token",
data=params,
headers=headers,
)
assert response.ok
token = response.json()["access_token"]
synth = SynthAPI(host=HAZY_HUB_HOST, api_key=token)
synth.train(cfg=..., project_id=...)